Navigating the Bare Metal: A Comprehensive Guide on How to Start Programming in Assembly Language
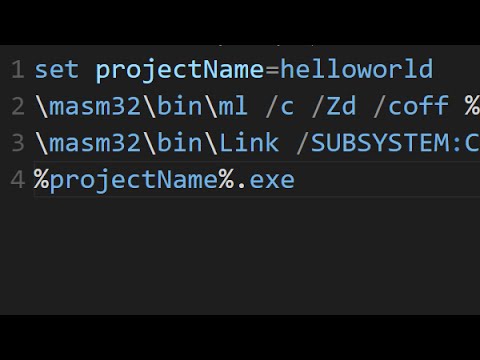
Introduction:
Programming in Assembly language is a journey into the heart of computing, where the code directly communicates with the underlying hardware. While often considered low-level, mastering Assembly offers unparalleled control and insight into a computer’s operations. In this extensive guide, we will embark on the path of learning Assembly programming, exploring its foundations, tools, and techniques. Whether you are a curious beginner or a seasoned developer, this guide aims to demystify Assembly and empower you to write efficient and optimized code at the closest proximity to the machine.
Section 1: Understanding Assembly Language
1.1 What is Assembly Language?
- Assembly language is a low-level programming language that provides a symbolic representation of machine code instructions.
- Each Assembly instruction corresponds to a specific operation that the computer’s central processing unit (CPU) can execute.
1.2 Why Learn Assembly?
- Gain a deep understanding of how computers work at the hardware level.
- Write highly efficient and optimized code for specific tasks.
- Develop insights that transcend higher-level programming languages.
Section 2: The Basics of Assembly Language
2.1 Assembly Instruction Format:
- Assembly instructions typically consist of an operation code (opcode) and operands.
- Operands can be registers, memory addresses, or immediate values.
2.2 Registers:
- Registers are small, fast storage locations within the CPU.
- Learn about general-purpose registers, special-purpose registers, and their roles in Assembly programming.
2.3 Memory and Addressing Modes:
- Understand how Assembly interacts with memory.
- Explore various addressing modes such as direct, indirect, and indexed addressing.
Section 3: Assembly Language Tools
3.1 Choosing an Assembly Language:
- Assembly languages are architecture-specific. Choose an Assembly language based on the architecture you want to work with (x86, ARM, MIPS, etc.).
3.2 Development Environments:
- Explore Assembly development environments like NASM (Netwide Assembler) for x86 architecture, GAS (GNU Assembler), or Keil for ARM architecture.
3.3 Emulators and Simulators:
- Use emulators or simulators like QEMU or Bochs to run Assembly programs in a controlled environment.
Section 4: Writing Your First Assembly Program
4.1 Setting Up Your Development Environment:
- Install the necessary tools and configure your development environment.
4.2 Writing a Simple Assembly Program:
- Create a basic Assembly program that prints “Hello, Assembly!” to the console.
- Understand the structure of an Assembly program, including sections like .text, .data, and .bss.
4.3 Assembling and Linking:
- Use the assembler (e.g., NASM) to convert Assembly code into machine code (object file) and the linker to create an executable file.
4.4 Running Your Program:
- Execute your Assembly program using an emulator or simulator.
Section 5: Assembly Language Programming Concepts
5.1 Control Structures:
- Explore control flow structures like jumps, loops, and conditional branches in Assembly.
- Understand how Assembly implements if-else statements and loops.
5.2 Procedures and Functions:
- Learn how to define and call procedures (functions) in Assembly.
- Understand the concept of the stack and its role in function calls.
5.3 Input and Output:
- Handle input and output operations in Assembly, including reading from the keyboard and writing to the console.
Section 6: Advanced Assembly Programming
6.1 Interrupts and System Calls:
- Understand how interrupts and system calls work in Assembly.
- Explore the use of interrupts for handling hardware events.
6.2 Bit Manipulation:
- Learn bitwise operations and bit manipulation techniques in Assembly.
- Understand the significance of flags and status registers.
6.3 Memory Management:
- Explore memory segmentation, allocation, and deallocation in Assembly.
- Understand the role of the Memory Management Unit (MMU).
Section 7: Debugging and Optimizing Assembly Code
7.1 Debugging Tools:
- Use debugging tools like GDB (GNU Debugger) to analyze and debug Assembly code.
- Explore features such as setting breakpoints, inspecting registers, and stepping through code.
7.2 Profiling and Optimization:
- Learn techniques for profiling Assembly code to identify performance bottlenecks.
- Explore optimization strategies for writing efficient Assembly programs.
Section 8: Resources and Further Learning
8.1 Books and Documentation:
- Refer to books like “Programming from the Ground Up” by Jonathan Bartlett and official documentation for the Assembly language and architecture you are working with.
8.2 Online Courses and Tutorials:
- Enroll in online courses and tutorials offered by platforms like Udemy, Coursera, or edX.
8.3 Community and Forums:
- Join Assembly language communities and forums to seek help, share knowledge, and connect with fellow enthusiasts.
Conclusion:
Programming in Assembly language is a journey into the inner workings of computers, offering a unique perspective on software development. This comprehensive guide has laid the foundation for understanding Assembly language, from its basics to advanced concepts. As you venture into the realm of Assembly programming, remember that practice, experimentation, and a curious mindset are key to mastering this powerful language. Embrace the challenges, celebrate the victories, and may your journey through Assembly programming be both enlightening and rewarding. Happy coding at the bare metal!