Mastering the Pythonic Art: A Comprehensive Guide on How to Start Programming in Python
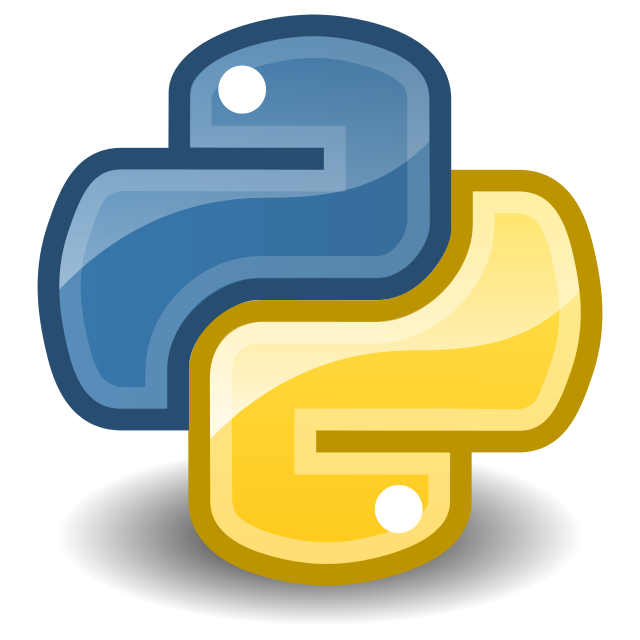
Introduction:
Python, a versatile and powerful programming language, has emerged as a favorite among beginners and experienced developers alike. Renowned for its readability, simplicity, and vast ecosystem of libraries, Python is an ideal language for those venturing into the world of programming. In this exhaustive guide, we will unravel the steps and concepts to embark on your journey of programming in Python. From setting up your development environment to mastering essential programming constructs, this guide aims to equip you with the knowledge and skills to dive into Python programming with confidence.
Section 1: Understanding the Basics of Python
1.1 What is Python?
- Python is a high-level, interpreted programming language known for its readability and ease of use.
- Guido van Rossum created Python, and it emphasizes code readability, allowing developers to express concepts in fewer lines of code.
1.2 Why Choose Python?
- Python’s simplicity and readability make it an excellent choice for beginners.
- A vast standard library and a strong community contribute to its popularity.
- Python is versatile, used in web development, data science, artificial intelligence, automation, and more.
Section 2: Setting Up Your Python Development Environment
2.1 Installing Python:
- Visit the official Python website (https://www.python.org/) to download the latest version of Python.
- Follow the installation instructions for your operating system.
2.2 Verifying Python Installation:
- Open a command prompt or terminal and type “python –version” to confirm that Python is installed successfully.
- Ensure that the Python executable is added to your system’s PATH variable.
2.3 Choosing a Code Editor or IDE:
- Select a code editor or integrated development environment (IDE) for writing Python code.
- Popular choices include Visual Studio Code, PyCharm, Jupyter Notebooks, and IDLE (included with Python).
Section 3: Writing Your First Python Program
3.1 Opening Your Code Editor:
- Open your chosen code editor or IDE to create a new Python file.
3.2 Hello, World!:
- Write a simple “Hello, World!” program to ensure that your development environment is set up correctly.pythonCopy code
print("Hello, World!")
3.3 Saving and Running Your Program:
- Save your Python file with a “.py” extension.
- Run the program by executing the script or using the command “python filename.py” in the terminal.
Section 4: Python Language Fundamentals
4.1 Variables and Data Types:
- Understand how to declare variables and explore fundamental data types (integers, floats, strings, booleans).
- Learn about dynamic typing and how Python infers variable types.
4.2 Control Flow Statements:
- Master control flow statements such as if, elif, and else for decision-making.
- Explore loop constructs like for and while for iterative tasks.
4.3 Functions:
- Grasp the concept of functions, defining them with the “def” keyword.
- Understand parameters, return values, and the importance of modular code.
Section 5: Python Data Structures
5.1 Lists:
- Learn about lists, a versatile data structure that stores an ordered collection of items.
- Explore list operations, slicing, and common list methods.
5.2 Dictionaries:
- Understand dictionaries, a key-value pair data structure.
- Explore dictionary operations and methods.
5.3 Sets:
- Learn about sets, an unordered collection of unique items.
- Explore set operations and methods.
Section 6: Object-Oriented Programming (OOP) in Python
6.1 Classes and Objects:
- Grasp the fundamentals of object-oriented programming (OOP) by creating classes and objects.
- Understand attributes and methods within classes.
6.2 Inheritance and Polymorphism:
- Explore inheritance to create subclasses and polymorphism to allow objects to take on multiple forms.
- Understand the concept of encapsulation.
Section 7: Python Modules and Libraries
7.1 Importing Modules:
- Learn how to use built-in modules and import external modules to extend Python’s functionality.
7.2 Exploring Popular Libraries:
- Explore popular Python libraries, including NumPy for numerical computing, pandas for data manipulation, and Matplotlib for data visualization.
Section 8: Handling Exceptions and Errors
8.1 Understanding Exceptions:
- Familiarize yourself with exceptions and how Python handles errors.
- Learn to use try, except, else, and finally blocks for error handling.
8.2 Writing Robust Code:
- Implement practices to write robust and error-tolerant Python code.
Section 9: Exploring Advanced Python Concepts
9.1 File Handling:
- Learn how to read from and write to files using Python.
- Explore different file modes and methods.
9.2 Regular Expressions:
- Understand regular expressions for pattern matching and text manipulation.
9.3 Multithreading and Multiprocessing:
- Explore concurrent programming using multithreading and multiprocessing in Python.
Section 10: Continuous Learning and Resources
10.1 Online Courses and Tutorials: – Enroll in online courses and tutorials offered by platforms like Coursera, Udacity, and edX.
10.2 Documentation and Books: – Refer to the official Python documentation (https://docs.python.org/) and explore books like “Python Crash Course” and “Fluent Python.”
10.3 Coding Challenges and Projects: – Engage in coding challenges on platforms like HackerRank and LeetCode. – Undertake personal projects to apply your skills and build a portfolio.
Conclusion:
Embarking on the journey of Python programming is an exciting endeavor that opens the doors to a myriad of possibilities. By following this comprehensive guide, you’ve gained insights into the basics of Python, set up your development environment, and explored fundamental concepts and advanced topics. Remember that learning to program is a continuous journey, and the Python community is vast and supportive. Use the resources provided, participate in coding communities, and embrace the joy of creating with Python. Happy coding!