Performing Unit Testing and Validation in LabVIEW
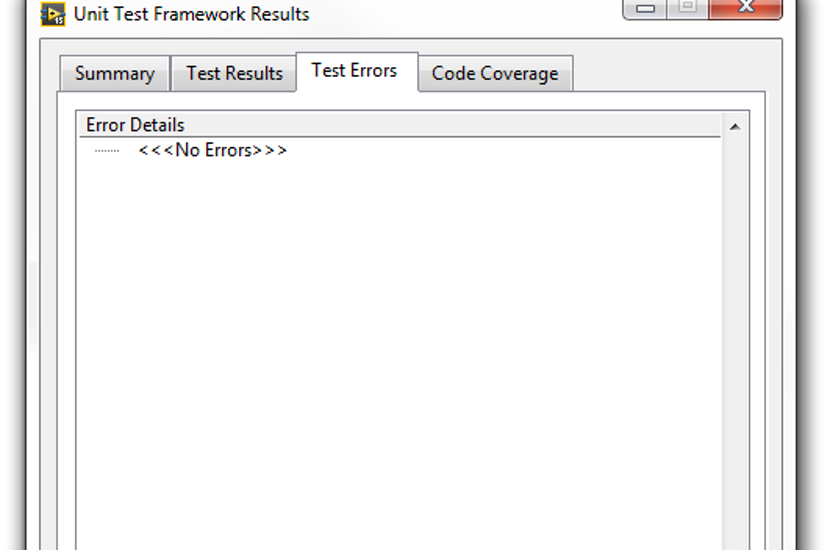
Introduction
Unit testing and validation are critical components of software development, ensuring that individual components and the overall system perform as expected. LabVIEW, a graphical programming language developed by National Instruments, is widely used for data acquisition, instrument control, and automation. Implementing rigorous unit testing and validation processes in LabVIEW applications is essential for delivering reliable, high-quality software. This comprehensive article will explore the principles, methodologies, and best practices for performing unit testing and validation in LabVIEW.
1. Fundamentals of Unit Testing
1.1. What is Unit Testing?
Unit testing involves testing individual units or components of a software application to verify that each unit performs as expected. A unit is the smallest testable part of an application, such as a function, subroutine, or method. Unit tests are typically automated and are written and executed by software developers during the development process.
1.2. Benefits of Unit Testing
Unit testing offers several key benefits:
- Early Bug Detection: Identifies defects early in the development process, reducing the cost and effort required to fix them.
- Code Quality: Improves code quality by enforcing modular and testable design practices.
- Documentation: Serves as documentation for the code, illustrating how each unit is expected to function.
- Refactoring: Facilitates code refactoring by ensuring that changes do not introduce new defects.
- Confidence: Increases developer confidence in the correctness and reliability of the code.
1.3. Unit Testing Principles
Effective unit testing adheres to several key principles:
- Isolation: Each unit test should test a single unit in isolation, without dependencies on other units or external systems.
- Repeatability: Unit tests should be repeatable and produce consistent results each time they are run.
- Automation: Unit tests should be automated to enable frequent and efficient execution.
- Independence: Unit tests should be independent of each other, ensuring that the outcome of one test does not affect others.
- Simplicity: Unit tests should be simple and focused, testing only the functionality of the unit under test.
2. Introduction to LabVIEW
2.1. What is LabVIEW?
LabVIEW (Laboratory Virtual Instrument Engineering Workbench) is a graphical programming environment developed by National Instruments. It is widely used for developing applications in the fields of data acquisition, instrument control, and automation. LabVIEW programs are called Virtual Instruments (VIs) and consist of a block diagram (code) and a front panel (user interface).
2.2. Key Features of LabVIEW
LabVIEW offers several features that make it well-suited for developing and testing software applications:
- Graphical Programming: Uses a dataflow programming model with intuitive block diagrams.
- Extensive Libraries: Provides a wide range of libraries for data acquisition, signal processing, and control.
- Hardware Integration: Seamlessly integrates with various hardware devices for data acquisition and control.
- Real-Time Processing: Supports real-time processing and deterministic control for time-critical applications.
- Modularity: Encourages modular design, making it easier to develop and test individual components.
2.3. LabVIEW Unit Testing Tools
LabVIEW provides several tools and frameworks for unit testing and validation:
- VI Tester: A unit testing framework for LabVIEW that supports automated testing and reporting.
- Unit Test Framework Toolkit: An add-on toolkit that provides tools for creating, managing, and executing unit tests.
- JKI VI Tester: An open-source unit testing framework that integrates with the LabVIEW development environment.
- Custom Test VIs: Developers can create custom VIs for unit testing, leveraging LabVIEW’s built-in functionality and libraries.
3. Setting Up the LabVIEW Environment for Unit Testing
3.1. Installing Necessary Tools and Toolkits
To perform unit testing in LabVIEW, you need to install the necessary tools and toolkits:
- LabVIEW Development Environment: Ensure you have the latest version of LabVIEW installed.
- VI Tester: Download and install the VI Tester framework from the NI Tools Network or relevant repositories.
- Unit Test Framework Toolkit: Install the Unit Test Framework Toolkit from National Instruments, which may require a separate license.
- JKI VI Tester: Download and install the JKI VI Tester from the JKI website or GitHub repository.
3.2. Setting Up a Project Structure
A well-organized project structure is essential for effective unit testing. Consider the following guidelines:
- Project Organization: Organize your project into folders and subfolders based on functionality, such as “Modules,” “Tests,” and “Utilities.”
- Test Folders: Create separate folders for unit tests corresponding to each module or component in your project.
- Naming Conventions: Use consistent naming conventions for VIs and test cases to make them easily identifiable.
- Version Control: Use a version control system (e.g., Git) to manage your project files and track changes.
3.3. Configuring the Development Environment
Configure the LabVIEW development environment to support unit testing:
- Paths and Dependencies: Ensure all necessary paths and dependencies are correctly configured in your project.
- LabVIEW Options: Adjust LabVIEW options to facilitate unit testing, such as enabling debugging and setting appropriate execution settings.
- Build Specifications: Define build specifications for your project, including targets for unit tests and application deployment.
4. Writing Unit Tests in LabVIEW
4.1. Identifying Units to Test
Identify the units to be tested in your LabVIEW application. Units typically include:
- Functions: Individual VIs that perform specific functions or calculations.
- SubVIs: Modular components used within larger VIs.
- Algorithms: Specific algorithms or processes implemented in VIs.
- Interfaces: Interfaces for interacting with external systems or hardware.
4.2. Creating Test Cases
Create test cases for each unit to verify its functionality. Follow these steps:
- Define Test Objectives: Clearly define the objectives of each test case, specifying what functionality is being tested.
- Input Parameters: Identify the input parameters required for the test case.
- Expected Output: Define the expected output or behavior for the given input parameters.
- Test Procedures: Outline the procedures for executing the test case and verifying the results.
4.3. Implementing Test VIs
Implement test VIs to execute the test cases and verify the results. Consider the following guidelines:
- Setup and Teardown: Implement setup and teardown procedures to initialize and clean up the test environment before and after each test case.
- Assertions: Use assertions to verify that the actual output matches the expected output. LabVIEW provides built-in functions for assertions, such as “Check Equals” and “Check True.”
- Test Data: Use representative test data that covers different scenarios and edge cases.
- Modularity: Keep test VIs modular and focused on testing specific units.
4.4. Running Unit Tests
Run the unit tests to verify the functionality of the units under test. Follow these steps:
- Execute Tests: Use the unit testing framework (e.g., VI Tester or Unit Test Framework Toolkit) to execute the test cases.
- Analyze Results: Analyze the test results to identify any failures or discrepancies.
- Debugging: Debug and fix any issues identified during testing.
- Re-running Tests: Re-run the tests after fixing issues to ensure that the units perform as expected.
5. Validation in LabVIEW
5.1. What is Validation?
Validation ensures that the overall system meets the specified requirements and performs its intended function. It involves verifying that the system works correctly in the real-world environment and meets user needs and expectations.
5.2. Importance of Validation
Validation is crucial for ensuring the reliability and effectiveness of the system:
- Compliance: Ensures compliance with industry standards and regulatory requirements.
- User Satisfaction: Ensures that the system meets user needs and expectations.
- Safety and Reliability: Ensures the safety and reliability of the system in real-world conditions.
- Performance: Verifies that the system performs efficiently and effectively.
5.3. Validation Processes
Validation involves several processes, including:
- Requirements Validation: Verifying that the system meets the specified requirements.
- Functional Validation: Verifying that the system performs the intended functions.
- Performance Validation: Verifying that the system meets performance criteria, such as response time and throughput.
- User Validation: Verifying that the system meets user needs and expectations through user acceptance testing (UAT).
6. Validation Techniques in LabVIEW
6.1. Simulation and Modeling
Simulation and modeling techniques can be used to validate system behavior under different conditions:
- Simulink Integration: Integrate LabVIEW with MATLAB/Simulink to perform system-level simulations and validate control algorithms.
- Hardware-in-the-Loop (HIL): Use HIL simulations to validate control systems by simulating real-world conditions and hardware interactions.
- System Models: Develop system models in LabVIEW to simulate and validate the behavior of individual components and the overall system.
6.2. Test Automation
Automating validation tests improves efficiency and repeatability:
- Automated Test Scripts: Develop automated test scripts in LabVIEW to perform validation tests.
- Test Sequencing: Use LabVIEW’s test sequencing capabilities to automate the execution of multiple test cases in a defined order.
- Data Logging: Implement data logging to capture and analyze test results automatically.
6.3. Hardware Testing
Hardware testing involves validating the system’s interaction with hardware components:
- Real-Time Testing: Perform real-time testing to validate the system’s behavior under real-world conditions.
- Integration Testing: Validate the integration of the system with external hardware devices and interfaces.
- Environmental Testing: Perform environmental testing to validate the system’s performance under different environmental conditions, such as temperature and humidity.
6.4. User Acceptance Testing (UAT)
User acceptance testing involves validating the system with end-users to ensure it meets their needs and expectations:
- User Scenarios: Develop user scenarios that represent real-world use cases.
- User Feedback: Collect feedback from users to identify any issues or areas for improvement.
- Acceptance Criteria: Define acceptance criteria based on user requirements and expectations.
7. Best Practices for Unit Testing and Validation in LabVIEW
7.1. Test-Driven Development (TDD)
Test-driven development (TDD) is a software development methodology that emphasizes writing tests before writing the actual code. Implementing TDD in LabVIEW involves the following steps:
- Write a Test Case: Write a test case for the desired functionality before implementing the code.
- Run the Test: Run the test case, which will initially fail since the code is not yet implemented.
- Write the Code: Implement the code to pass the test case.
- Run the Test Again: Run the test case again to verify that the code passes the test.
- Refactor: Refactor the code as necessary to improve quality and maintainability, ensuring that the test continues to pass.
7.2. Continuous Integration (CI)
Continuous integration (CI) is a practice that involves integrating code changes frequently and running automated tests to detect issues early. Implementing CI in LabVIEW involves:
- CI Tools: Use CI tools such as Jenkins, GitLab CI, or TeamCity to automate the build and test process.
- Automated Tests: Ensure that unit tests and validation tests are automated and included in the CI pipeline.
- Frequent Commits: Commit code changes frequently to the version control repository.
- Build Triggers: Configure build triggers to automatically run tests whenever code changes are committed.
7.3. Code Reviews and Peer Testing
Code reviews and peer testing involve reviewing and testing code written by colleagues to identify defects and improve code quality:
- Code Reviews: Conduct regular code reviews to identify issues, improve code quality, and share knowledge.
- Peer Testing: Have peers review and test your code to provide additional perspectives and catch issues that you might have missed.
7.4. Documentation and Reporting
Documentation and reporting are essential for maintaining a comprehensive record of tests and their results:
- Test Documentation: Document test cases, test procedures, and expected results for reference and reproducibility.
- Test Reports: Generate test reports that summarize test results, including any failures or issues identified.
- Traceability: Ensure traceability between test cases and requirements to verify that all requirements are tested and validated.
8. Case Studies and Applications
8.1. Medical Devices
Medical device development requires rigorous testing and validation to ensure safety and compliance with regulatory standards:
- Unit Testing: Perform unit testing of individual components, such as signal processing algorithms and control loops.
- Validation: Validate the overall system through simulations, hardware testing, and user acceptance testing.
- Compliance: Ensure compliance with industry standards, such as ISO 13485 and FDA regulations.
8.2. Automotive Systems
Automotive systems require reliable and efficient testing and validation processes:
- Unit Testing: Test individual components, such as engine control units (ECUs) and sensor interfaces.
- HIL Testing: Use hardware-in-the-loop (HIL) simulations to validate control algorithms and system behavior.
- Performance Validation: Validate system performance under different driving conditions and environments.
8.3. Industrial Automation
Industrial automation systems require thorough testing and validation to ensure reliable and efficient operation:
- Unit Testing: Test individual components, such as control algorithms and communication interfaces.
- Integration Testing: Validate the integration of the system with external devices and networks.
- Real-Time Testing: Perform real-time testing to validate the system’s performance and behavior under real-world conditions.
8.4. Aerospace and Defense
Aerospace and defense systems require stringent testing and validation to ensure safety and reliability:
- Unit Testing: Test individual components, such as navigation algorithms and communication systems.
- Simulation and Modeling: Use simulations and modeling to validate system behavior and performance.
- Environmental Testing: Perform environmental testing to validate the system’s performance under different conditions, such as temperature, pressure, and vibration.
9. Challenges and Solutions
9.1. Challenges
Developing and validating LabVIEW applications presents several challenges:
- Complexity: Industrial and scientific applications can be complex, with many interconnected components and systems.
- Real-Time Requirements: Ensuring real-time performance and deterministic behavior can be challenging.
- Integration: Integrating LabVIEW with external systems, devices, and protocols can be difficult.
- Scalability: Scaling the system to handle large volumes of data and multiple devices.
- Security: Ensuring the security of the system against cyber threats and unauthorized access.
9.2. Solutions
To overcome these challenges, consider the following solutions:
- Modular Design: Design the system in a modular fashion to simplify development, testing, and maintenance.
- Test Automation: Automate testing processes to improve efficiency and repeatability.
- Simulation and Modeling: Use simulation and modeling to validate system behavior under different conditions.
- Integration Planning: Plan the integration of LabVIEW with external systems and devices from the beginning.
- Security Measures: Implement robust security measures to protect the system against cyber threats.
10. Future Trends
10.1. AI and Machine Learning
AI and machine learning are increasingly being used to enhance testing and validation processes. LabVIEW’s integration with AI and machine learning libraries enables the development of intelligent testing solutions that can:
- Predict Failures: Use predictive analytics to identify potential failures before they occur.
- Optimize Testing: Use machine learning algorithms to optimize test procedures and improve efficiency.
- Automate Analysis: Automate the analysis of test results to identify patterns and anomalies.
10.2. Cloud-Based Testing
Cloud-based testing offers several advantages for LabVIEW applications:
- Scalability: Leverage cloud resources to scale testing processes and handle large volumes of data.
- Accessibility: Enable remote access to testing environments and resources.
- Collaboration: Facilitate collaboration between distributed teams by providing a centralized testing platform.
10.3. Enhanced Security
Security is becoming increasingly important in testing and validation processes. Future trends include:
- Secure Development Practices: Implementing secure development practices to ensure the integrity and security of the code.
- Secure Testing Environments: Using secure testing environments to protect sensitive data and prevent unauthorized access.
- Cybersecurity Testing: Performing cybersecurity testing to identify and mitigate vulnerabilities in the system.
10.4. Real-Time Data Analytics
Real-time data analytics is becoming more prevalent in testing and validation processes:
- Real-Time Monitoring: Implement real-time monitoring of test processes and results.
- Data Visualization: Use advanced data visualization tools to analyze and interpret test data.
- Automated Decision-Making: Implement automated decision-making processes based on real-time data analysis.
Conclusion
Performing unit testing and validation in LabVIEW is essential for developing reliable, high-quality software applications. By leveraging LabVIEW’s graphical programming capabilities, extensive libraries, and testing tools, developers can create robust unit tests and validation processes that ensure the functionality, performance, and reliability of their applications. This comprehensive guide has covered the principles, methodologies, and best practices for unit testing and validation in LabVIEW, providing developers with the knowledge and tools needed to succeed. By staying abreast of emerging trends and technologies, developers can continue to enhance their testing and validation processes, paving the way for innovative and intelligent solutions in various industries.