Mastering Python Classes: A Comprehensive Guide
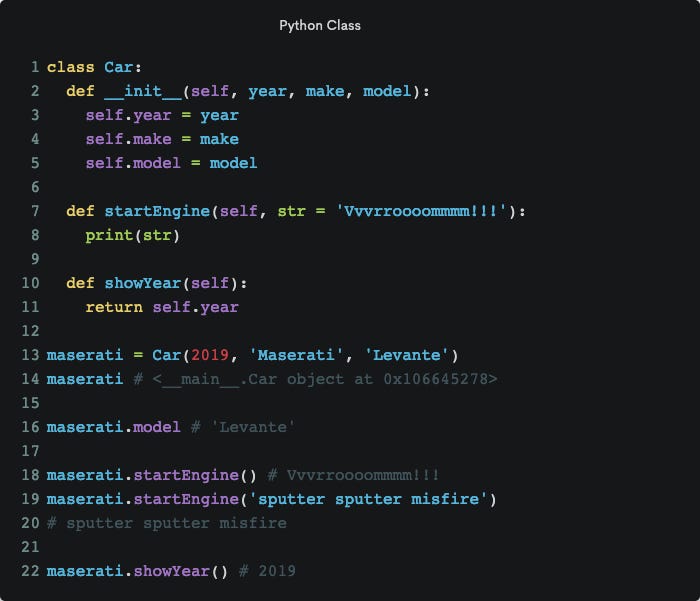
Understanding Classes and Objects
Before delving into the intricacies of Python classes, let’s establish a foundational understanding.
What is a Class? A class is a blueprint or template for creating objects. It defines the properties (attributes) and behaviors (methods) that objects of that class will have. Think of a class as a cookie cutter: it defines the shape of the cookie, but the actual cookies are the objects created from that cutter.
What is an Object? An object is an instance of a class. It is a concrete realization of the blueprint defined by the class. Each object has its own set of data (attributes) and can perform actions (methods) as defined by the class.
Creating a Class
To create a class in Python, use the class
keyword followed by the class name and a colon.
Python
class Dog:
pass
Use code with caution.
The pass
keyword is a placeholder, indicating that the class body is empty for now.
Class Attributes and Methods
Attributes are variables that belong to a class. They can be accessed by all instances of the class.
Python
class Dog:
species = 'Canis lupus familiaris'
Use code with caution.
Methods are functions defined within a class. They operate on the object’s data.
Python
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print(f"{self.name} barks!")
Use code with caution.
The __init__
Method
The __init__
method is a special method called the constructor. It is automatically called when an object of the class is created. It is used to initialize the object’s attributes.
Creating Objects (Instantiation)
To create an object of a class, you call the class name as if it were a function.
Python
my_dog = Dog("Buddy", "Golden Retriever")
Use code with caution.
Accessing Attributes and Methods
You can access an object’s attributes and call its methods using the dot notation.
Python
print(my_dog.name) # Output: Buddy
my_dog.bark() # Output: Buddy barks!
Use code with caution.
Class and Instance Variables
- Class variables: Shared by all instances of a class.
- Instance variables: Unique to each instance of a class.
Python
class Dog:
species = 'Canis lupus familiaris' # Class variable
def __init__(self, name, breed):
self.name = name # Instance variable
self.breed = breed # Instance variable
Use code with caution.
Inheritance
Inheritance allows you to create new classes that inherit attributes and methods from existing classes.
Python
class Mammal:
def walk(self):
print("Walking")
class Dog(Mammal):
pass
my_dog = Dog()
my_dog.walk() # Output: Walking
Use code with caution.
Polymorphism
Polymorphism means that objects of different types can be treated as if they were of the same type.
Python
def make_sound(animal):
animal.make_sound()
class Dog:
def make_sound(self):
print("Woof!")
class Cat:
def make_sound(self):
print("Meow!")
dog = Dog()
cat = Cat()
make_sound(dog) # Output: Woof!
make_sound(cat) # Output: Meow!
Use code with caution.
Encapsulation
Encapsulation is the bundling of data (attributes) and methods that operate on that data within a single unit (class). It helps protect data from accidental modification and improves code organization.
Abstraction
Abstraction focuses on the essential features of an object, hiding unnecessary details. Abstract classes are blueprints for other classes and cannot be instantiated.
Python
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
Use code with caution.
Special Methods
Python provides several special methods that can be overridden to customize object behavior. Some common ones include:
__str__()
: Returns a string representation of the object.__repr__()
: Returns a string representation suitable for debugging.__len__()
: Returns the length of the object.__iter__()
: Returns an iterator for the object.__getitem__()
: Defines how elements are accessed using indexing.
Conclusion
Python classes are a fundamental concept in object-oriented programming. By understanding classes, objects, attributes, methods, inheritance, polymorphism, encapsulation, and abstraction, you can write cleaner, more organized, and reusable code.