Mastering Matplotlib: A Comprehensive Guide to Data Visualization
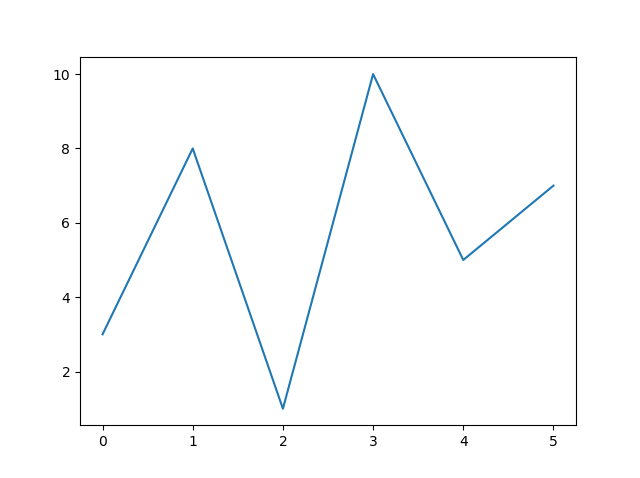
Introduction to Matplotlib
Matplotlib is a powerful Python library for creating static, animated, and interactive visualizations. It offers a wide range of plot types, customization options, and integration capabilities. This comprehensive guide will explore the fundamentals of Matplotlib and delve into various plotting techniques.
Getting Started with Matplotlib
To begin using Matplotlib, import the necessary modules:
Python
import matplotlib.pyplot as plt
import numpy as np
The pyplot
module provides a MATLAB-like interface for creating plots. NumPy is often used for generating data to be visualized.
Basic Plotting
Line Plots
A line plot is suitable for visualizing trends and patterns in data over a continuous interval.
Python
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('sin(x)')
plt.title('Sine Curve')
plt.show()
Scatter Plots
Scatter plots are useful for visualizing relationships between two numerical variables.
Python
x = np.random.rand(100)
y = np.random.rand(100)
plt.scatter(x, y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Scatter Plot')
plt.show()
Bar Plots
Bar plots are effective for comparing categorical data.
Python
categories = ['A', 'B', 'C', 'D']
values = [25, 35, 20, 40]
plt.bar(categories, values)
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot')
plt.show()
Histograms
Histograms provide a visual representation of the distribution of numerical data.
Python
data = np.random.randn(1000)
plt.hist(data, bins=30)
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram')
plt.show()
Customizing Plots
Matplotlib offers extensive customization options to tailor plots to specific needs.
- Line styles:
plt.plot(x, y, linestyle='--')
- Marker styles:
plt.scatter(x, y, marker='^')
- Colors:
plt.plot(x, y, color='red')
- Labels:
plt.xlabel('x'), plt.ylabel('y'), plt.title('Title')
- Legends:
plt.legend()
- Grids:
plt.grid(True)
- Axis limits:
plt.xlim(), plt.ylim()
- Subplots:
plt.subplot(nrows, ncols, plot_number)
Advanced Plotting Techniques
- Error bars:
plt.errorbar(x, y, yerr=error)
- Box plots:
plt.boxplot(data)
- Pie charts:
plt.pie(data)
- Contour plots:
plt.contour(X, Y, Z)
- 3D plots:
import matplotlib.pyplot as plt
- Animations:
import matplotlib.animation as animation
Matplotlib Object-Oriented Interface
For more complex visualizations and customization, Matplotlib provides an object-oriented interface.
Python
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot(x, y)
ax.set_xlabel('x')
ax.set_ylabel('y')
ax.set_title('Line Plot')
plt.show()
Saving Plots
To save a plot as an image file, use plt.savefig('filename.png')
.
Conclusion
Matplotlib is a versatile library for creating a wide range of plots. By mastering its fundamentals and exploring advanced techniques, you can effectively visualize and communicate data insights.