How to Use Map, Filter, and Reduce in JavaScript
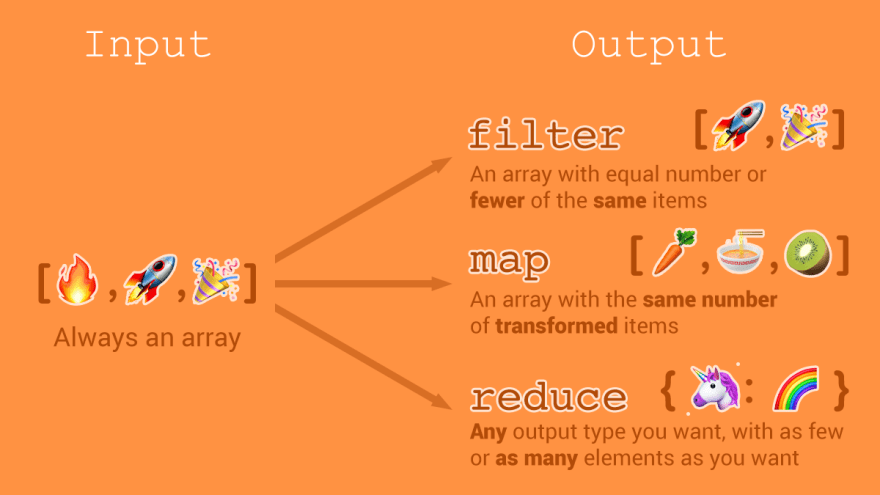
JavaScript provides a variety of array methods that make manipulating and processing arrays much easier. Among these methods, map
, filter
, and reduce
are particularly powerful. They allow you to transform, filter, and aggregate data in concise and readable ways. Understanding these methods is crucial for writing clean, efficient, and functional JavaScript code.
Table of Contents
- Introduction to Array Methods
- Understanding
map
- What is
map
? - Syntax
- Examples
- Use Cases
- What is
- Understanding
filter
- What is
filter
? - Syntax
- Examples
- Use Cases
- What is
- Understanding
reduce
- What is
reduce
? - Syntax
- Examples
- Use Cases
- What is
- Combining
map
,filter
, andreduce
- Practical Examples
- Performance Considerations
- Best Practices
- Conclusion
Introduction to Array Methods
Arrays in JavaScript come with built-in methods that allow you to perform common operations in a functional programming style. The map
, filter
, and reduce
methods are part of the ECMAScript 5 (ES5) specification and are widely supported in modern JavaScript environments.
These methods are higher-order functions, meaning they take other functions as arguments. This enables you to write concise and expressive code that performs complex operations on arrays.
Understanding map
What is map
?
The map
method creates a new array populated with the results of calling a provided function on every element in the calling array. It is commonly used to transform each element of an array.
Syntax
javascript
Copy code
let newArray = array.map(function(currentValue, index, array) { // Return element for newArray });
currentValue
: The current element being processed in the array.index
(Optional): The index of the current element being processed in the array.array
(Optional): The arraymap
was called upon.
Examples
Basic Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let squaredNumbers = numbers.map(function(number) { return number * number; }); console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Using Arrow Functions
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let squaredNumbers = numbers.map(number => number * number); console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Mapping Objects
javascript
Copy code
let users = [ { firstName: "John", lastName: "Doe" }, { firstName: "Jane", lastName: "Smith" }, { firstName: "Emily", lastName: "Jones" } ]; let fullNames = users.map(user => `${user.firstName} ${user.lastName}`); console.log(fullNames); // Output: ["John Doe", "Jane Smith", "Emily Jones"]
Use Cases
- Transforming Data: When you need to apply a function to each element in an array to produce a new array.
- Extracting Values: When you need to create a new array based on a specific property of objects in the original array.
- Formatting Data: When you need to format data elements in an array to match a specific output.
Understanding filter
What is filter
?
The filter
method creates a new array with all elements that pass the test implemented by the provided function. It is commonly used to remove unwanted elements from an array.
Syntax
javascript
Copy code
let newArray = array.filter(function(currentValue, index, array) { // Return true to keep the element, false otherwise });
currentValue
: The current element being processed in the array.index
(Optional): The index of the current element being processed in the array.array
(Optional): The arrayfilter
was called upon.
Examples
Basic Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let evenNumbers = numbers.filter(function(number) { return number % 2 === 0; }); console.log(evenNumbers); // Output: [2, 4]
Using Arrow Functions
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let evenNumbers = numbers.filter(number => number % 2 === 0); console.log(evenNumbers); // Output: [2, 4]
Filtering Objects
javascript
Copy code
let users = [ { name: "John", age: 25 }, { name: "Jane", age: 30 }, { name: "Emily", age: 22 } ]; let adults = users.filter(user => user.age >= 25); console.log(adults); // Output: [{ name: "John", age: 25 }, { name: "Jane", age: 30 }]
Use Cases
- Removing Elements: When you need to remove certain elements from an array based on a condition.
- Finding Matches: When you need to find all elements in an array that match a certain criteria.
- Creating Subsets: When you need to create a subset of an array based on specific conditions.
Understanding reduce
What is reduce
?
The reduce
method executes a reducer function (that you provide) on each element of the array, resulting in a single output value. It is commonly used for accumulating values or computing a single result from an array.
Syntax
javascript
Copy code
let result = array.reduce(function(accumulator, currentValue, index, array) { // Return value to be passed to the next iteration }, initialValue);
accumulator
: The accumulator accumulates the callback’s return values.currentValue
: The current element being processed in the array.index
(Optional): The index of the current element being processed in the array.array
(Optional): The arrayreduce
was called upon.initialValue
(Optional): A value to use as the first argument to the first call of the callback.
Examples
Summing an Array
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let sum = numbers.reduce(function(accumulator, number) { return accumulator + number; }, 0); console.log(sum); // Output: 15
Using Arrow Functions
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let sum = numbers.reduce((accumulator, number) => accumulator + number, 0); console.log(sum); // Output: 15
Calculating Average
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let average = numbers.reduce((accumulator, number, index, array) => { accumulator += number; if (index === array.length - 1) { return accumulator / array.length; } return accumulator; }, 0); console.log(average); // Output: 3
Flattening an Array
javascript
Copy code
let arrays = [[1, 2], [3, 4], [5, 6]]; let flattened = arrays.reduce((accumulator, array) => accumulator.concat(array), []); console.log(flattened); // Output: [1, 2, 3, 4, 5, 6]
Use Cases
- Accumulating Values: When you need to accumulate or combine all elements of an array into a single value.
- Transforming Data: When you need to transform an array into a single value, such as an object or another array.
- Performing Complex Calculations: When you need to perform complex calculations or aggregations on array elements.
Combining map
, filter
, and reduce
Practical Examples
Combining map
, filter
, and reduce
allows you to perform complex data transformations and manipulations in a concise and readable way.
Example 1: Filtering and Mapping
javascript
Copy code
let users = [ { name: "John", age: 25 }, { name: "Jane", age: 30 }, { name: "Emily", age: 22 } ]; let adultNames = users .filter(user => user.age >= 25) .map(user => user.name); console.log(adultNames); // Output: ["John", "Jane"]
Example 2: Filtering, Mapping, and Reducing
javascript
Copy code
let transactions = [ { type: "income", amount: 100 }, { type: "expense", amount: 50 }, { type: "income", amount: 150 }, { type: "expense", amount: 30 } ]; let totalIncome = transactions .filter(transaction => transaction.type === "income") .map(transaction => transaction.amount) .reduce((accumulator, amount) => accumulator + amount, 0); console.log(totalIncome); // Output: 250
Example 3: Complex Data Transformation
javascript
Copy code
let orders = [ { product: "Laptop", quantity: 1, price: 1000 }, { product: "Mouse", quantity