How to Loop Through Arrays in JavaScript
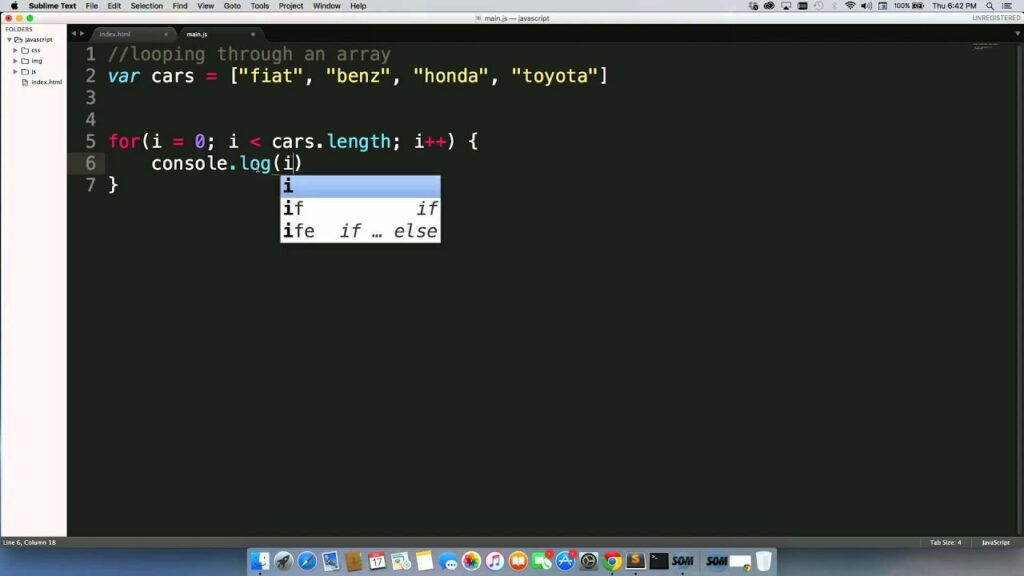
Arrays are a fundamental data structure in JavaScript, providing a way to store multiple values in a single variable. One of the most common operations performed on arrays is looping through their elements. JavaScript offers various methods and techniques for iterating over arrays, each with its own use cases and benefits.
In this comprehensive guide, we will explore the different ways to loop through arrays in JavaScript, including traditional loops, higher-order functions, and modern ES6+ methods. We’ll cover their syntax, usage, examples, and best practices.
Table of Contents
- Introduction to Arrays
- Traditional Loops
for
Loopwhile
Loopdo...while
Loop
- ES6+ Methods
for...of
LoopforEach
Methodmap
Methodfilter
Methodreduce
Methodevery
andsome
Methods
- Additional Looping Techniques
for...in
Loop- Using
forEach
with Arrow Functions
- Performance Considerations
- Best Practices for Looping Through Arrays
- Conclusion
Introduction to Arrays
Arrays in JavaScript are used to store multiple values in a single variable. These values can be of any data type, including numbers, strings, objects, and even other arrays.
Creating Arrays
You can create arrays in JavaScript using square brackets []
or the Array
constructor.
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let fruits = ["apple", "banana", "cherry"]; let mixedArray = [1, "hello", true, { key: "value" }, [1, 2, 3]]; let newArray = new Array(1, 2, 3, 4, 5);
Accessing Array Elements
Array elements are accessed using their index, which starts from 0.
javascript
Copy code
console.log(numbers[0]); // Output: 1 console.log(fruits[2]); // Output: cherry console.log(mixedArray[3]); // Output: { key: "value" }
Traditional Loops
for
Loop
The for
loop is the most commonly used loop for iterating over arrays. It consists of three parts: initialization, condition, and increment/decrement.
Syntax
javascript
Copy code
for (initialization; condition; increment) { // Code to be executed }
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; for (let i = 0; i < numbers.length; i++) { console.log(numbers[i]); }
while
Loop
The while
loop executes a block of code as long as a specified condition is true.
Syntax
javascript
Copy code
while (condition) { // Code to be executed }
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let i = 0; while (i < numbers.length) { console.log(numbers[i]); i++; }
do...while
Loop
The do...while
loop is similar to the while
loop, but it guarantees that the code block is executed at least once.
Syntax
javascript
Copy code
do { // Code to be executed } while (condition);
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let i = 0; do { console.log(numbers[i]); i++; } while (i < numbers.length);
ES6+ Methods
for...of
Loop
The for...of
loop, introduced in ES6, is a modern way to iterate over iterable objects, including arrays.
Syntax
javascript
Copy code
for (variable of iterable) { // Code to be executed }
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; for (let number of numbers) { console.log(number); }
forEach
Method
The forEach
method executes a provided function once for each array element.
Syntax
javascript
Copy code
array.forEach(function(currentValue, index, array) { // Code to be executed });
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; numbers.forEach(function(number) { console.log(number); });
map
Method
The map
method creates a new array with the results of calling a provided function on every element in the calling array.
Syntax
javascript
Copy code
let newArray = array.map(function(currentValue, index, array) { // Return element for newArray });
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let squaredNumbers = numbers.map(function(number) { return number * number; }); console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
filter
Method
The filter
method creates a new array with all elements that pass the test implemented by the provided function.
Syntax
javascript
Copy code
let newArray = array.filter(function(currentValue, index, array) { // Return true to keep the element, false otherwise });
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let evenNumbers = numbers.filter(function(number) { return number % 2 === 0; }); console.log(evenNumbers); // Output: [2, 4]
reduce
Method
The reduce
method executes a reducer function (that you provide) on each element of the array, resulting in a single output value.
Syntax
javascript
Copy code
let result = array.reduce(function(accumulator, currentValue, index, array) { // Return value to be passed to the next iteration }, initialValue);
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let sum = numbers.reduce(function(accumulator, number) { return accumulator + number; }, 0); console.log(sum); // Output: 15
every
and some
Methods
The every
method tests whether all elements in the array pass the test implemented by the provided function. The some
method tests whether at least one element in the array passes the test implemented by the provided function.
Syntax
javascript
Copy code
let allPass = array.every(function(currentValue, index, array) { // Return true if all elements pass the test, false otherwise }); let somePass = array.some(function(currentValue, index, array) { // Return true if at least one element passes the test, false otherwise });
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let allEven = numbers.every(function(number) { return number % 2 === 0; }); console.log(allEven); // Output: false let someEven = numbers.some(function(number) { return number % 2 === 0; }); console.log(someEven); // Output: true
Additional Looping Techniques
for...in
Loop
The for...in
loop iterates over the enumerable properties of an object. It is generally not recommended for arrays because it iterates over the properties, not the elements, and may include properties inherited from the prototype chain.
Syntax
javascript
Copy code
for (variable in object) { // Code to be executed }
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; for (let index in numbers) { console.log(numbers[index]); }
Using forEach
with Arrow Functions
Arrow functions provide a more concise syntax for anonymous functions and are often used with forEach
.
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; numbers.forEach(number => console.log(number));
Performance Considerations
When looping through large arrays, performance can become an issue. Here are some tips to optimize your loops:
- Minimize Loop Overhead: Use simple loops (
for
orwhile
) for large arrays to minimize overhead. - Cache Array Length: Store the array length in a variable to avoid recalculating it in each iteration.
- Use Efficient Methods: Use methods like
map
,filter
, andreduce
for concise and efficient operations.
Example
javascript
Copy code
let numbers = [1, 2, 3, 4, 5]; let length = numbers.length; for (let i = 0; i < length; i++) { console.log(numbers[i]); }
Best Practices for Looping Through Arrays
- Choose the Right Loop: Use
for
loops for traditional iteration,forEach
for simple operations, andmap
,filter
, orreduce
for functional programming. - Use Arrow Functions: Prefer arrow functions for concise and readable code.
- Avoid
for...in
for Arrays: Usefor...of
or other methods to iterate over array elements. - Keep Code Readable: Write clear and understandable code, even if it means sacrificing a bit of performance.
- Avoid Modifying Arrays Inside Loops: Modifying the array you’re looping through can lead to unexpected behavior and bugs.
Conclusion
Looping through arrays is a fundamental skill in JavaScript programming. Whether you are using traditional loops or modern ES6+ methods, understanding how to iterate over arrays efficiently and effectively is crucial. Each looping method has its own strengths and use cases, and choosing the right one can make your code more readable, maintainable, and performant.
By following best practices and considering performance implications, you can ensure that your array operations are optimized and error-free. Keep experimenting with different methods, and continue to deepen your understanding of JavaScript and its powerful array-handling capabilities.