How to Handle Missing Data and NaN Values in MATLAB
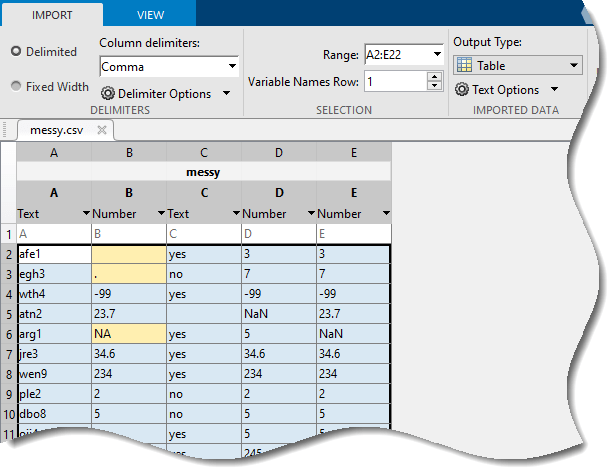
Handling missing data and NaN (Not a Number) values is a crucial aspect of data analysis and preprocessing. In MATLAB, various functions and techniques are available to effectively manage, analyze, and clean datasets containing missing or undefined values. This comprehensive article explores the different methods and strategies for handling missing data and NaN values in MATLAB.
Table of Contents
- Introduction to Missing Data and NaN Values
- Identifying Missing Data and NaN Values
- Using
isnan
- Using
ismissing
- Using
- Creating Missing Data and NaN Values
- Removing Missing Data and NaN Values
- Removing Rows or Columns
- Removing Specific Elements
- Replacing Missing Data and NaN Values
- Replacing with Specific Values
- Replacing with Interpolated Values
- Replacing with Mean, Median, or Mode
- Handling Missing Data in Tables
- Detecting Missing Data
- Removing Missing Data
- Replacing Missing Data
- Handling Missing Data in Time Series
- Interpolation Methods
- Filling Gaps in Time Series Data
- Advanced Techniques for Handling Missing Data
- Imputation Methods
- Machine Learning Approaches
- Practical Applications and Examples
- Data Cleaning and Preprocessing
- Statistical Analysis
- Machine Learning and Predictive Modeling
- Best Practices and Tips
- Conclusion
1. Introduction to Missing Data and NaN Values
Missing data occurs when certain values are absent from a dataset. These missing values can arise due to various reasons such as data entry errors, sensor malfunctions, or incomplete data collection. In MATLAB, missing values are often represented as NaN (Not a Number) or can be marked using specific placeholders like NaT
for datetime arrays.
Handling missing data is essential for ensuring the accuracy and reliability of data analysis and modeling. Unaddressed missing values can lead to biased results, reduced statistical power, and misleading conclusions.
2. Identifying Missing Data and NaN Values
Using isnan
The isnan
function is used to identify NaN values in arrays and matrices. It returns a logical array of the same size as the input, with true
values indicating the presence of NaN.
matlab
Copy code
% Create an array with NaN values A = [1, 2, NaN, 4, NaN]; % Identify NaN values nan_mask = isnan(A); % Display the result disp(nan_mask);
Using ismissing
The ismissing
function is a more general tool for detecting missing data in arrays, tables, and cell arrays. It identifies missing values based on a specified criteria or default missing values for the data type.
matlab
Copy code
% Create a table with missing values T = table([1; 2; NaN], {'A'; 'B'; ''}, 'VariableNames', {'Value', 'Category'}); % Identify missing values missing_mask = ismissing(T); % Display the result disp(missing_mask);
3. Creating Missing Data and NaN Values
Missing data can be intentionally introduced into datasets for testing or simulation purposes.
matlab
Copy code
% Create a 5x5 matrix B = magic(5); % Introduce NaN values B(2, 3) = NaN; B(4, 5) = NaN; % Display the matrix with NaN values disp(B);
4. Removing Missing Data and NaN Values
Removing Rows or Columns
Rows or columns containing missing values can be removed using the rmmissing
function.
matlab
Copy code
% Create a matrix with NaN values C = [1, 2, NaN; 4, NaN, 6; 7, 8, 9]; % Remove rows with NaN values C_cleaned_rows = rmmissing(C); % Remove columns with NaN values C_cleaned_cols = rmmissing(C, 2); % Display the results disp(C_cleaned_rows); disp(C_cleaned_cols);
Removing Specific Elements
Individual elements containing NaN can be removed or filtered out using logical indexing.
matlab
Copy code
% Create an array with NaN values D = [1, 2, NaN, 4, NaN, 6]; % Remove NaN values D_cleaned = D(~isnan(D)); % Display the result disp(D_cleaned);
5. Replacing Missing Data and NaN Values
Replacing with Specific Values
Missing values can be replaced with specific values using the fillmissing
function.
matlab
Copy code
% Create a matrix with NaN values E = [1, 2, NaN; 4, NaN, 6; 7, 8, 9]; % Replace NaN values with zero E_filled = fillmissing(E, 'constant', 0); % Display the result disp(E_filled);
Replacing with Interpolated Values
Interpolation can be used to estimate missing values based on surrounding data points.
matlab
Copy code
% Create an array with NaN values F = [1, 2, NaN, 4, 5, NaN, 7]; % Replace NaN values with linear interpolation F_interpolated = fillmissing(F, 'linear'); % Display the result disp(F_interpolated);
Replacing with Mean, Median, or Mode
Missing values can be replaced with summary statistics such as the mean, median, or mode.
matlab
Copy code
% Create an array with NaN values G = [1, 2, NaN, 4, NaN, 6]; % Replace NaN values with the mean G_filled_mean = fillmissing(G, 'movmean', 3); % Replace NaN values with the median G_filled_median = fillmissing(G, 'movmedian', 3); % Display the results disp(G_filled_mean); disp(G_filled_median);
6. Handling Missing Data in Tables
Detecting Missing Data
Missing data in tables can be detected using the ismissing
function.
matlab
Copy code
% Create a table with missing values T = table([1; NaN; 3], {'A'; ''; 'C'}, 'VariableNames', {'Value', 'Category'}); % Identify missing values missing_mask_table = ismissing(T); % Display the result disp(missing_mask_table);
Removing Missing Data
Rows or columns with missing data in tables can be removed using the rmmissing
function.
matlab
Copy code
% Remove rows with missing values T_cleaned_rows = rmmissing(T); % Remove columns with missing values T_cleaned_cols = rmmissing(T, 2); % Display the results disp(T_cleaned_rows); disp(T_cleaned_cols);
Replacing Missing Data
Missing values in tables can be replaced using the fillmissing
function.
matlab
Copy code
% Replace missing values with the previous value T_filled_prev = fillmissing(T, 'previous'); % Replace missing values with the next value T_filled_next = fillmissing(T, 'next'); % Display the results disp(T_filled_prev); disp(T_filled_next);
7. Handling Missing Data in Time Series
Interpolation Methods
Interpolation methods can be used to estimate missing values in time series data.
matlab
Copy code
% Create a time series with missing values time = datetime(2021,1,1) + days(0:5); values = [1, NaN, 3, NaN, 5, 6]; ts = timetable(time', values'); % Interpolate missing values ts_interpolated = retime(ts, 'daily', 'linear'); % Display the result disp(ts_interpolated);
Filling Gaps in Time Series Data
Gaps in time series data can be filled using various methods such as forward filling or backward filling.
matlab
Copy code
% Forward fill missing values ts_filled_forward = fillmissing(ts, 'previous'); % Backward fill missing values ts_filled_backward = fillmissing(ts, 'next'); % Display the results disp(ts_filled_forward); disp(ts_filled_backward);
8. Advanced Techniques for Handling Missing Data
Imputation Methods
Advanced imputation methods, such as k-nearest neighbors (KNN) or multiple imputation, can be used to handle missing data.
matlab
Copy code
% KNN imputation example using 'knnimpute' function from the Bioinformatics Toolbox data = [1, 2, NaN; 4, NaN, 6; 7, 8, 9]; % Perform KNN imputation data_imputed = knnimpute(data); % Display the result disp(data_imputed);
Machine Learning Approaches
Machine learning models can be trained to predict and impute missing values.
matlab
Copy code
% Load a dataset with missing values load fisheriris; data = meas; data(1:10, 3) = NaN; % Introduce missing values % Train a regression model to predict missing values mdl = fitlm(data(:, [1, 2, 4]), data(:, 3)); % Predict missing values predicted_values = predict(mdl, data(:, [1, 2, 4])); % Impute missing values data_imputed_ml = data; data_imputed_ml(1:10, 3) = predicted_values(1:10); % Display the result disp(data_imputed_ml);
9. Practical Applications and Examples
Data Cleaning and Preprocessing
Handling missing data is a critical step in data cleaning and preprocessing pipelines.
matlab
Copy code
% Load a dataset with missing values data = readtable('data_with_missing.csv'); % Remove rows with missing values data_cleaned = rmmissing(data); % Replace missing values with the mean data_filled = fillmissing(data, 'constant', mean(data.Value, 'omitnan')); % Display the cleaned and filled data disp(data_cleaned); disp(data_filled);
Statistical Analysis
Accurate statistical analysis requires handling missing data appropriately.
matlab
Copy code
% Create a dataset with missing values data = [1, 2, NaN, 4, NaN, 6]; % Compute the mean, excluding NaN values mean_value = mean(data, 'omitnan'); % Compute the standard deviation, excluding NaN values std_value = std(data, 'omitnan'); % Display the results disp(mean_value); disp(std_value);
Machine Learning and Predictive Modeling
Handling missing data is crucial for building robust machine learning models.
matlab
Copy code
% Load a dataset with missing values data = readtable('data_with_missing.csv'); % Impute missing values using KNN data_filled_knn = knnimpute(table2array(data)); % Convert the filled data back to a table data_filled_knn = array2table(data_filled_knn, 'VariableNames', data.Properties.VariableNames); % Train a machine learning model using the imputed data mdl = fitctree(data_filled_knn, 'Target'); % Display the trained model view(mdl, 'Mode', 'graph');
10. Best Practices and Tips
- Understand the Nature of Missing Data: Determine whether the missing data is missing completely at random (MCAR), missing at random (MAR), or missing not at random (MNAR), as this will influence the handling method.
- Visualize Missing Data: Use visualizations such as heatmaps or bar plots to understand the distribution and pattern of missing values.
- Choose Appropriate Imputation Methods: Select imputation methods that are suitable for the data type and the specific analysis or modeling task.
- Evaluate Imputation Quality: Assess the quality of imputed values by comparing with known data, if available, or by validating the results through cross-validation.
- Document and Report: Clearly document the methods used for handling missing data and report any potential biases or limitations introduced by these methods.
11. Conclusion
Handling missing data and NaN values is a fundamental aspect of data analysis and preprocessing in MATLAB. With a variety of functions and techniques available, MATLAB provides powerful tools to identify, remove, and replace missing values, ensuring the integrity and reliability of data analysis and modeling. By understanding and applying the appropriate methods, users can effectively manage missing data and improve the quality of their analyses and predictive models.