How to Create and Manipulate Arrays and Matrices in MATLAB
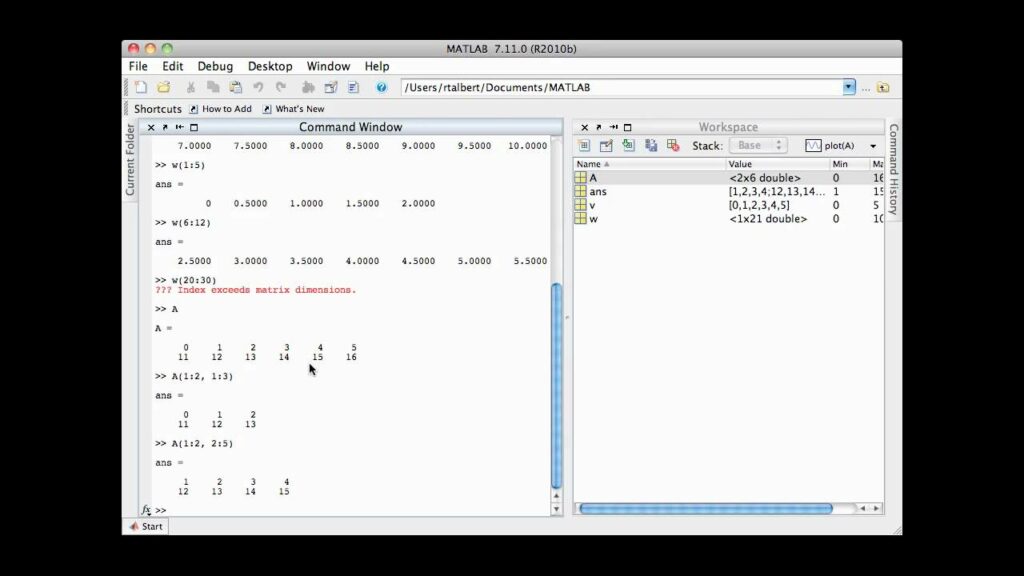
Arrays and matrices form the foundation of many mathematical computations in MATLAB, a powerful tool used extensively in engineering, science, and mathematics. This article provides an in-depth guide on how to create, manipulate, and perform operations on arrays and matrices in MATLAB.
Table of Contents
- Introduction to Arrays and Matrices in MATLAB
- Creating Arrays and Matrices
- Basic Methods
- Special Matrices
- Preallocating Arrays
- Indexing and Slicing Arrays
- Basic Operations on Arrays and Matrices
- Arithmetic Operations
- Element-wise Operations
- Matrix Operations
- Advanced Manipulations
- Reshaping Arrays
- Concatenation
- Permutations and Transpositions
- Working with Sparse Matrices
- Linear Algebra with Matrices
- Solving Systems of Linear Equations
- Eigenvalues and Eigenvectors
- Singular Value Decomposition
- Practical Applications
- Data Analysis
- Image Processing
- Signal Processing
- Optimization and Performance Tips
- Conclusion
1. Introduction to Arrays and Matrices in MATLAB
MATLAB, which stands for “Matrix Laboratory,” is designed for matrix computations. Arrays and matrices are essential data structures in MATLAB, enabling users to store and manipulate data efficiently. MATLAB supports various types of arrays, including numerical arrays, logical arrays, character arrays, and cell arrays.
2. Creating Arrays and Matrices
Basic Methods
Row and Column Vectors
Creating row and column vectors is straightforward in MATLAB.
matlab
Copy code
% Row vector row_vector = [1, 2, 3, 4, 5]; % Column vector column_vector = [1; 2; 3; 4; 5];
Matrices
Matrices can be created using square brackets, with semicolons separating the rows.
matlab
Copy code
% 2x3 matrix matrix = [1, 2, 3; 4, 5, 6];
Using Functions
MATLAB provides functions to create arrays and matrices.
matlab
Copy code
% Create a 3x3 matrix of zeros zero_matrix = zeros(3, 3); % Create a 2x2 matrix of ones one_matrix = ones(2, 2); % Create a 4x4 identity matrix identity_matrix = eye(4); % Create a 1x5 vector with values from 1 to 5 vector = 1:5; % Create a 1x5 vector with values from 1 to 5 with a step of 0.5 vector_step = 1:0.5:5; % Create a 1x100 vector with linearly spaced values between 0 and 1 linspace_vector = linspace(0, 1, 100); % Create a 1x100 vector with logarithmically spaced values between 1 and 100 logspace_vector = logspace(0, 2, 100);
Special Matrices
MATLAB provides functions to create special matrices useful in various applications.
matlab
Copy code
% Create a 3x3 magic square magic_square = magic(3); % Create a 4x4 matrix of random numbers uniformly distributed between 0 and 1 random_matrix = rand(4, 4); % Create a 3x3 matrix of normally distributed random numbers randn_matrix = randn(3, 3); % Create a Hilbert matrix of size 5x5 hilbert_matrix = hilb(5);
Preallocating Arrays
Preallocating memory for arrays is important for optimizing performance, especially in loops.
matlab
Copy code
% Preallocate a 10x10 matrix of zeros preallocated_matrix = zeros(10, 10); % Example: Using preallocated array in a loop for i = 1:10 preallocated_matrix(i, :) = i * ones(1, 10); end
3. Indexing and Slicing Arrays
MATLAB uses one-based indexing, and elements can be accessed using parentheses.
matlab
Copy code
% Create a 3x3 matrix A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % Access the element in the second row, third column element = A(2, 3); % Access the entire second row second_row = A(2, :); % Access the entire third column third_column = A(:, 3); % Access a submatrix (rows 1 to 2, columns 2 to 3) submatrix = A(1:2, 2:3);
4. Basic Operations on Arrays and Matrices
Arithmetic Operations
MATLAB supports standard arithmetic operations on arrays and matrices.
matlab
Copy code
% Define two matrices A = [1, 2; 3, 4]; B = [5, 6; 7, 8]; % Addition C = A + B; % Subtraction D = A - B; % Matrix multiplication E = A * B; % Scalar multiplication F = 2 * A; % Matrix power G = A^2;
Element-wise Operations
Element-wise operations are performed using the dot (.) operator.
matlab
Copy code
% Element-wise multiplication H = A .* B; % Element-wise division I = A ./ B; % Element-wise power J = A .^ 2;
Matrix Operations
MATLAB provides functions for common matrix operations.
matlab
Copy code
% Transpose A_transpose = A'; % Inverse A_inverse = inv(A); % Determinant A_determinant = det(A); % Rank A_rank = rank(A); % Trace A_trace = trace(A);
5. Advanced Manipulations
Reshaping Arrays
Arrays can be reshaped using the reshape
function.
matlab
Copy code
% Create a 2x6 matrix A = [1, 2, 3, 4, 5, 6; 7, 8, 9, 10, 11, 12]; % Reshape into a 3x4 matrix B = reshape(A, [3, 4]);
Concatenation
Arrays can be concatenated horizontally or vertically using square brackets.
matlab
Copy code
% Define two matrices A = [1, 2; 3, 4]; B = [5, 6; 7, 8]; % Horizontal concatenation C = [A, B]; % Vertical concatenation D = [A; B];
Permutations and Transpositions
Arrays can be permuted or transposed to reorder their dimensions.
matlab
Copy code
% Define a 3x2x2 array A = cat(3, [1, 2; 3, 4], [5, 6; 7, 8]); % Permute dimensions B = permute(A, [2, 1, 3]); % Transpose a 2D matrix C = A(:, :, 1)';
6. Working with Sparse Matrices
Sparse matrices store only non-zero elements, saving memory and computation time.
matlab
Copy code
% Create a sparse matrix S = sparse([1, 3, 1], [2, 3, 3], [10, 20, 30], 5, 5); % Convert a full matrix to sparse A = [1, 0, 0; 0, 2, 0; 0, 0, 3]; S = sparse(A); % Convert a sparse matrix to full A_full = full(S);
7. Linear Algebra with Matrices
Solving Systems of Linear Equations
MATLAB can solve systems of linear equations using the backslash operator (\
).
matlab
Copy code
% Define a system of equations Ax = b A = [1, 2; 3, 4]; b = [5; 6]; % Solve for x x = A \ b;
Eigenvalues and Eigenvectors
Eigenvalues and eigenvectors can be computed using the eig
function.
matlab
Copy code
% Define a matrix A = [1, 2; 2, 3]; % Compute eigenvalues and eigenvectors [V, D] = eig(A);
Singular Value Decomposition
Singular Value Decomposition (SVD) is computed using the svd
function.
matlab
Copy code
% Define a matrix A = [1, 2; 3, 4]; % Compute SVD [U, S, V] = svd(A);
8. Practical Applications
Data Analysis
Arrays and matrices are essential in data analysis for storing and manipulating datasets.
matlab
Copy code
% Load a dataset data = load('data.mat'); % Compute the mean of each column mean_values = mean(data); % Compute the standard deviation of each column std_values = std(data);
Image Processing
Images can be represented as matrices, with each element corresponding to a pixel value.
matlab
Copy code
% Read an image image = imread('image.jpg'); % Convert the image to grayscale gray_image = rgb2gray(image); % Apply a Gaussian filter filtered_image = imgaussfilt(gray_image, 2); % Display the original and filtered images subplot(1, 2, 1); imshow(gray_image); title('Original Image'); subplot(1, 2, 2); imshow(filtered_image); title('Filtered Image');
Signal Processing
MATLAB is widely used in signal processing for analyzing and manipulating signals.
matlab
Copy code
% Generate a signal t = 0:0.001:1; signal = sin(2 * pi * 50 * t) + sin(2 * pi * 120 * t); % Compute the Fourier transform signal_fft = fft(signal); % Plot the signal subplot(2, 1