Learn Python3 Full Course : Principles and Philosophy : Chapter 01
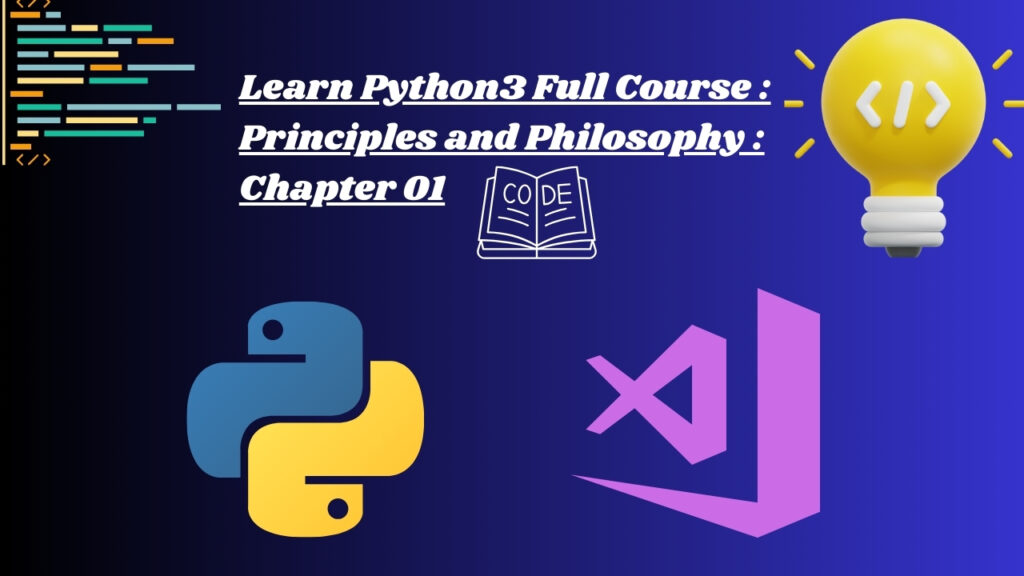
The Philosophy of Python
In the event that it appears to be odd to start a programming book with a part about philosophy, that is as a matter of fact
proof of why this part is so significant. Python was created to embody and enable a particular set
of principles that have guided the decisions of its maintainers and its community for nearly 20 years.
Understanding these concepts will help you make the most out of what the language and its community
have to offer.
Obviously, we’re not discussing Plato or Nietzsche here. Python deals with programming
problems, and its philosophies are intended to help build reliable, effective solutions. Some of these
philosophies are officially written into the Python landscape, while others are guidelines widely
accepted by Python programmers, but all of them will help you write code that is robust, easy to
maintain, and understandable to other developers.
The philosophies laid out in this part can be read from beginning to end here, but don’t expect to
commit them all to memory in one pass. The rest of this book will refer back here often, by showing
which concepts come into play in various situations. After all, the real value of philosophy is
understanding how to apply it when it matters most.
The Zen of Python
Perhaps the most famous collection of Python philosophy was written by Tim Peters, long-time
contributor to the language and its newsgroup, comp.lang.python. This Zen of Python combines some
of the most well-known philosophical concerns into a short list that has been recorded as both its own Python
Enhancement Proposal (PEP) and within Python itself. Something of a hidden treat, Python includes a
module called this.
>>> import this The Zen of Python, by Tim Peters Beautiful is better than ugly. Explicit is better than implicit. Simple is better than complex. Complex is better than complicated. Flat is better than nested. Sparse is better than dense. Readability counts. Special cases aren't special enough to break the rules. Although practicality beats purity. Errors should never pass silently. Unless explicitly silenced. In the face of ambiguity, refuse the temptation to guess. There should be one-- and preferably only one --obvious way to do it. Although that way may not be obvious at first unless you're Dutch. Now is better than never. Although never is often better than *right* now. If the implementation is hard to explain, it's a bad idea. If the implementation is easy to explain, it may be a good idea. Namespaces are one honking great idea -- let's do more of those!
This list was originally intended as a humorous accounting of Python philosophy, but over the years,
many Python applications have used these guidelines to greatly improve the quality, readability, and
effectiveness of their code. Simply listing the Zen of Python is of little value, though, so the following
sections will explain each maxim in more detail.
Beautiful Is Better Than Ugly
Perhaps it’s fitting that this first idea is arguably the most subjective of the whole bunch. After all,
beauty is in the eye of the beholder, a fact which has been discussed for centuries. It serves as an absolute
reminder that philosophy is far from absolute. However, having something like this in writing provides a goal
to strive for, which is the ultimate purpose of all these precepts.
One obvious application of this philosophy is in Python’s own syntax, which minimizes
the use of punctuation, instead favoring English words where appropriate. Another benefit is
Python’s focus on keyword arguments, which help clarify function calls that would be difficult, indeed
to understand. Consider the following two possible ways of writing the same code, and consider which
one looks more beautiful.
is_valid = form != null && form.is_valid(true)
is_valid = form is not None and form.is_valid(include_hidden_fields=True)
The second example reads a bit more like plain English, and explicitly including the name of the
argument gives greater insight into its purpose. In addition to language concerns, coding style can be
influenced by similar ideas of beauty. The name is_valid
, for example, asks a simple question, which
the method can then be expected to answer with its return value. A name like validate
would’ve been
questionable because it would be an accurate name even if no value were returned at all.
Being so subjective, however, relying too heavily on beauty as a criterion for design is a dangerous
decision. If other ideals have been considered and you’re still left with two workable options, certainly
consider factoring beauty into the equation, but make sure that other aspects are considered
first. You’ll likely find a good choice using some of the other guidelines well before reaching this point.
Explicit Is Better Than Implicit
Though this concept may seem easier to interpret than beauty, it’s actually one of the trickier precepts
to follow. On the surface, it seems simple enough: do nothing the programmer didn’t explicitly
command. Beyond Python itself, frameworks and libraries have a similar duty because
their code will be accessed by other programmers whose goals won’t always be known in advance.
Unfortunately, truly explicit code must account for every nuance of a program’s execution, from
memory management to display routines. Some programming languages do expect that level of detail
from their developers, but Python does not. To make the programmer’s job easier and allow
you to focus on the task at hand, there must be some compromises along the way.
In general, Python asks that you declare your intentions explicitly, rather than issue every command
necessary to make that intention a reality. For example, when assigning a value to a variable, you don’t
have to worry about reserving the necessary memory, assigning a pointer to the value, and cleaning
up the memory once it’s no longer in use. Memory management is an essential part of variable
assignment, so Python takes care of it behind the scenes. Assigning the value is enough of an explicit
declaration of intent to justify the implicit behavior.
On the other hand, regular expressions in the Perl programming language automatically assign
values to special variables any time a match is found. Someone new to the way Perl handles that
situation wouldn’t understand a code snippet that relies on it because variables would seem to come
from thin air, with no assignments related to them. Python programmers try to avoid this kind of implicit
behavior for clearer code.
Since different applications will have different ways of declaring intentions, no single formal
explanation will apply to all cases. Instead, this precept will come up frequently throughout the
book, clarifying how it would be applied to various situations.